Comprehensive Overview of C++: Core Concepts, Advanced Features & Modern Applications
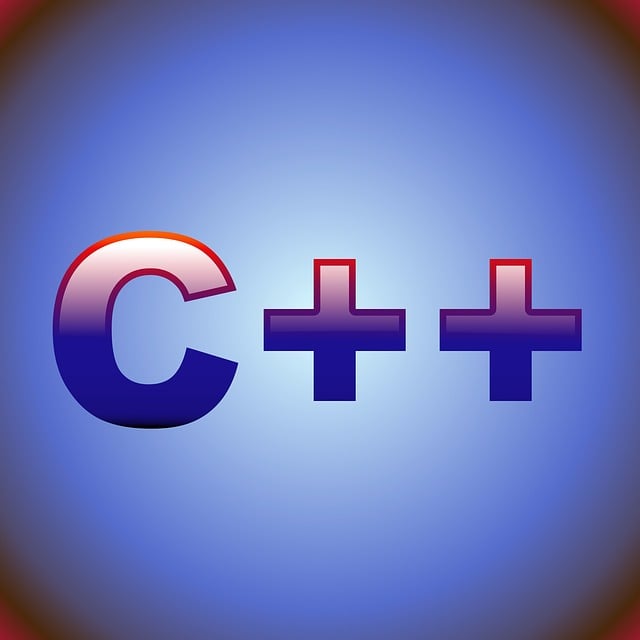
Overview of C++
It is a dynamo in the domain of programming languages. C++ was created by Bjarne Stroustrup in 1979 as an extension of the C programming language, merging together both efficiency and object-oriented programming. This powerhouse language is an extension of the C language in a way that maintains its efficiency within object-oriented programming.
C++ Features place it far ahead of the other languages. Developers like it since it can purvey both low-level memory manipulations and high-level abstractions. This is what makes the language very appealing and quite popular in the performance domain—it’s got a huge standard library.
C++ development
The transition from C to C++ marked a landmark move in the history of programming. Stroustrup had imagined that features could be added to C that would support object-oriented programming and still not lose its speed and efficiency. And hence it was “C with Classes,” which later became C++.
C++ has modernized a lot since then, and the language has seen many game-changing features added in and changed over the years through the addition of major versions, like C++98, C++11, and C++17. These changes have progressively polished and added to the language’s capabilities while preserving backward compatibility.
Core Concepts in C++
Object-Oriented Programming is at the very core of C++. It perfectly enables the developer to structure his code around objects holding data with behavior. Throughout Object-Oriented Programming, one may also invoke ease in modular, reusable, and maintainable coding.
Seriously, OOP is one of the chief backbones to C++, but it is no stranger to acting as a supportive language for procedural programming. This characteristic is inherited from C, where the developer can write out coding step by step. Apart from this, the blending of OOP and procedural programming allows flexibility in different styles of coding.
Another important concept of C++ is generic programming, in which coders have the flexibility to code using any type of data—a feature that gives more code reusability and type safety for making strong software very efficiently and flexibly.
Setting up the Development Environment
While coding with C++, choosing the correct IDE is very important. Some of the most popular are Visual Studio, Code::Blocks, and CLion. Each has several features that have something very special, useful, and unique to service different kinds of development needs and individual preferences.
The second thing involved in the setup of a C++ development environment is the installation of the compiler and tools. The generally used compilers are the GCC (GNU Compiler Collection) and Clang. Additionally, tools like CMake for build automation and Valgrind for memory debugging really boost the development.
Basic Syntax and Structure
C++ syntax extends that of C through to the provision of features for object-oriented and generic programming. Variables and data types form the base for a C++ program. C++ provides a large number of standard data types, including integers, floating-point numbers, and characters.
The operators and expressions available in C++ are very helpful in performing complex data manipulations. C++ offers a wide range of operators—from arithmetic expressions to bitwise manipulations. These sophisticated instruments derive programs through intricate algorithms and data structures.
Control structures in C++ exercise control and regulation over the flow of execution in a program. Conditional statements with if-else and switch-case bring decision-making capability to a C++ code. Looping constructs through for, while, and do-while enable one to do repetitive tasks, thereby allowing one to express more.
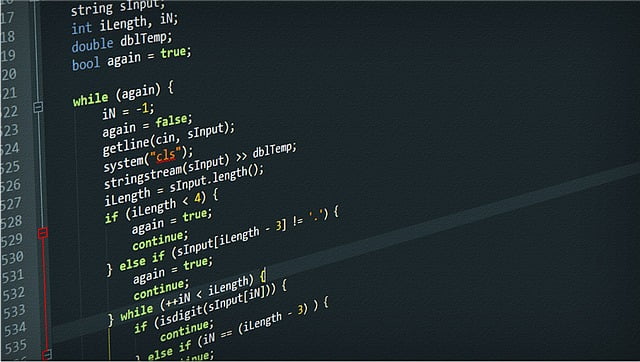
Functions in C++
Function declaration and definition are the most crucial parts of C++ programming. Functions encapsulate code, which is reusable, hence promote modularity and readability. C++ also recognizes other forms of functions, such as member functions and standalone functions.
Function overloading in C++ is a feature that allows defining multiple functions with the same name, but the quantity and type of passed parameters must be different. It enhances code readability, and one can design functions flexibly.
Another optimized performance technique of inline functions is that it’s one of the ways to minimize overhead that incurs during a function call. The functionality enhances the focus of C++ with regard to performance and efficiency.
Object-Oriented Programming in C++
Classes and objects outline the basis of OOP in C++. A class contains a layout that uses data members and member functions to describe an object. Objects are instances of classes, delivering life to the blueprints in memory.
Inheritance in C++ allows users to establish hierarchical relationships among classes. The inheritance mechanism leads to code reusability of the base class and modeling complex real-world relationships. Single, multiple, or multilevel inheritance is supported by C++, and it does not limit class design.
Another main feature of the OOP paradigm is polymorphism, which allows objects of different types to be treated uniformly. C++ supports polymorphism through virtual functions and function overriding. It provides flexibility and code extensibility.
C++ supports encapsulation using three key access specifiers: public, private, and protected. These three keywords are responsible for controlling visibility in class members and access to those members, maintaining data hiding, and therefore abstraction.
Memory Management
C++ gives the programmer very fine control of memory management. A programmer needs to know the difference between stack and heap memory to be effective when programming. Memory used by local variables lives on the stack and is managed automatically; otherwise, allocation and deallocation is done on the heap.
Dynamic memory allocation in C++ is done with the help of new and delete operators. These operators give runtime memory allocation, which helps create the data structures on the basis of the size defined during program execution. But manual memory management could also be a problem with things like a memory leak and a dangling pointer.
C++ introduced the concept of smart pointers to overcome challenges in memory management. A smart pointer is an object playing the role of a pointer but with additional functionalities, such as automatic memory deallocation. The smart pointers unique_ptr, shared_ptr, and weak_ptr significantly limit the risk of memory leaks and enhance the safety of code.
Templates and Generic Programming
The user can work with many data types from within a single function using function templates in C++. They provide the leverage of writing such code that has reusability and completeness in terms of types being taken. A unique template function can thus be written to handle a unique logic toward handling many types.
Class templates are a concept that comes with templates, but it relates to the class. They enable users to define generic classes that can work with multiple types of data. Class templates serve well in implementing data structures like linked lists and binary trees, which can store different data types.
The Standard Library Template, STL, is a very powerful collection of template classes and template functions. This is powerful because implementations for most of the common data structures and algorithms are provided, which in turn saves a lot of development time. STL’s backbone is formed of such containers like vectors, lists, and maps, and their algorithms for sorting and searching.
Exception Handling
Try-catch blocks form the basis of exception handling in C++. This mechanism allows the graceful handling of runtime errors. One is able to fit proper handling of errors by enclosing potentially error-prone code within a try block and trapping exceptions with a catch block.
A structured way to propagate error information is provided by throwing and catching exceptions, when independent parts of a program are strung together. In C++, almost an object of nearly any type can be thrown, particularly objects of user-defined exception classes. This generality permits detailed error reporting and handling.
It is also possible to create custom exception classes for representing particular error conditions and, hence, creating an exception hierarchy based on standard exception classes. This way, an improved level of error granularity will be galvanized. It also enhances the possibility of maintaining the code.
FILE HANDLING IN C++
Input/output streams in C++ present a common interface for dealing with diverse operations. The iostream library gives the functionality of file input using the ifstream and file output by using the ofstream classes. In helping with the diverse operations that take place in file handling, the classes ease the processes of reading and writing files.
File operations in C++ perform a plethora of functions. From opening files to closing files and reading to writing data, C++ has all-encompassing file I/O tools. Besides, it has some error-handling mechanisms that help perform robust file operations, even in cases like file-not-found-error or permission-denied error.
Serialization is the process in which objects are converted into a format suitable for storage or transmission. It is quite an advanced feature in file handling in C++. Libraries and implementations of techniques are done in which serialization could be implemented. This will be particularly useful to persist complex data structures and for inter-process communication.
Advanced C++ Features
A namespace in C++ is a declarative region, a place where you can define names into this region and give different meanings to different identifiers in several regions. It allows a developer to provide different contexts for names, subsequently improving code organization and readbility. A great example of how to put namespaces into play in C++ is demonstrated by the std namespace, where storage for standard library entities is specified.
This enables developers to define custom behaviors for operators when acting on user-defined types. This feature allows developers to define more natural interfaces for their classes and write the implementation of these classes in a way typical for builtin types. However, this may hurt the readability of the code and there are some surprises in store for the unwary reader.
Lambda expressions are a way to succinctly define an anonymous function object; they are new in C++11. They are very useful where one-off short functions are needed—for example, with algorithms that accept function objects as arguments. Improving code readability and, hence, decreasing a need for separate function definitions are the real values the lambda expressions provide.
Multithreading and Concurrency
It gives creation and control of the thread, so that programmer can make the program concurrent. C++11 introduced a new library known as and lt;thread& gt; for standard thread handling. It makes code to be able to run in parallel.
Synchronization mechanisms are responsible for relating accesses to shared resources in a multithreaded program. The main synchronization facilities provided by C++ are mutexes, condition variables, and atomic operations. These facilities prevent race conditions and guarantee the viability of threads in a concurrent code.
Atomic operations are the only way to make an indivisible read-modify-write operation in order to share variables. A developer is provided with atomic types and operations in the library of C++ and develops lock-free programming in some cases. These atomic operations are the basic tool to build efficient concurrent data structures and algorithms.
Best Practices and Coding Standards
Taking care of proper naming conventions enhances code readability and maintainability. A clearly defined naming strategy of variables, functions, and classes helps make the developer understand the structure and purpose of the code without much effort. Although concrete conventions may differ for various projects/organizations, it is all about achieving consistency with a particular codebase.
Code organization bears a lot of importance in handling large C++ projects. With proper structuring of header and source files, thoughtful use of namespaces, and modules, one can have a clean and manageable codebase. A well-organized structure of C++ code is not only helpful in maintaining a good codebase but also aids in onboarding new team members.
Performance optimization is one of the important features of C++ programming. These level optimization techniques, including copying redundancies, realizing move semantics, and exploiting compiler optimizations, may increase the efficiency of the program by several orders of magnitude. Optimization efforts must be balanced so that the efforts do not affect the readability and maintainability of the code.
Debugging and Testing
Debugging helps identify and troubleshoot problems within C++ programs. The debugger tools in IDEs, breakpoints, and watch variables are spread throughout the codes in order to do the needful. Some other key ideas that help in the betterment of one’s debugging process are the concept of stack traces and basic memory layouts.
Google Test and Catch2 are unit testing frameworks that provide excellent tools for automated test implementation on C++ projects. Comprehensive unit testing is one way of asserting correctness and supporting development through refactoring. Good test-driven development practice really helps in creating a much more reliable and maintainable code base.
Profiling tools are used to point out performance bottlenecks in a C++ program. Profiling is conducted using tools such as gprof and Callgrind from Valgrind. They perform analysis of how a program is run and, therefore, point to optimization hotspots. Profiling, then, is important in line with making sure that C++ programs meet performance estimations provided, notably under resource-constrained environments.
C++ in Modern Software Development
Game development is one such area where C++ has remained maintained. These characteristics of performance and low-level in its control make this language suitable during high-performance game engine development. A lot of popular game engines have C++ parts, including Unreal Engine and Unity.
C++ is also widely used in systems programming—developing, for example, an operating system at the kernel level or the writing of device drivers. The ability of the language to work directly with hardware, coupled with a high level of abstractions, makes C++ fit for writing quite large system-level programs.
Also, due to its efficiency and object-oriented strengths, C++ forms the basis for the development of embedded systems. C++ is applied to multiple embedded domains, from automotive systems to popular IoT devices. Its ability to support resource-constrained environments makes this language an all-time favorite in this domain.
Comparison with Other Languages
While Java enables platform-independent provision through its virtual machine, C++ has provided the provision for more direct control over the resources of a system. Generally, C++ is more performance-focused and Java is more focused on the security features as well as the standard library.
Python is famous for its ease and speed of development, while C++ is more complex with a focus on performance. C++ shines in computation-intensive activities; Python works best in such activities as data analysis and rapid prototyping. In practice, many projects use both languages concurrently: C++ for performance-critical components, and Python for high-level scripting.
Rust, a newer systems programming language, has some of the same goals as C++: where performance and low-level control are of the highest priority. However, Rust is more concerned with memory safety and data-race freedom, achieved irrespective of garbage collection. While C++ is considerably more mature in ecosystems and tools, Rust brings along strong guarantees against common programming errors.
Future of C++
The C++ language is continuing to evolve, with upcoming features concentrating on productivity and performance. Some of the highly anticipated additions are modules, coroutines, and better syntax for templates. All these promising features will make C++ even more expressive and easier to use without giving up its nature of being performance-oriented.
Evolving standards are being guided by the ISO C++ committee in order to maintain C++ in the mainstream, taking into consideration the technology landscape. The careful considerations of the committee in the introduction of modern features with concern for backward compatibility place C++ in a position to adapt to new programming paradigms and hardware architectures.
Learning Resources of C++
There are, of course, very many books or courses available online for learners at different levels of C++ proficiency, from “The C++ Programming Language” by Bjarne Stroustrup to online platforms such as Coursera and edX. Rehearsal sessions will be found on the interactive coding platforms HackerRank and LeetCode, to acquire real-world problem-solving experience.
Community forums and websites are invaluable adjuncts to any C++ developer. Websites like Stack Overflow and cplusplus.com provide platforms for posting queries and sharing information. Involvement with such communities not only helps the developer in problem-solving but also keeps him updated with best practices and emerging trends in C++ development.
Conclusion
C++ remains one of the best examples of a beautifully designed programming language. Efficiency, in concert with expressiveness and flexibility, is one of the principal reasons C++ is used primarily in a vast field of applications, from system-level programming to high-performance computing. These reasons keep C++ in the mainstream of software infrastructure.
The C++ programming language has matured with the consistent advancement in technology. It is poised to take the great promise and great burden of this immense scope of opportunities and challenges, and developers entering or already in the grand world of C++ programming find it quite challenging but exciting for learning and using in practice. Its insight is in basic programming and state-of-the-art techniques.